Vulkan Procedural Grass Rendering

For my Master's year at Sheffield Hallam University, I spent a semester working on my dissertation project consisting of a project and corresponding research using the scientific method. Following several rabbit holes and finding the area I wanted to innovate within, I settled on a procedural grass renderer using Vulkan. I had spent some time prior with the API to learn how it works and gain a deeper understanding of low-level systems. This research attempts to answer the question
"Can utilising Vulkan's full GPU capabilities improve the performance and visual fidelity of procedural grass rendering?"
focusing on a comparison between the low-level explicit GPU communication with Vulkan, and the high-level abstracted nature of Unity using this renderer as the application used to generate data for the research evaluation. This project provided me a much deeper understanding into advanced graphics and compute pipelines and how they work with shaders to create realistic and performant applications.
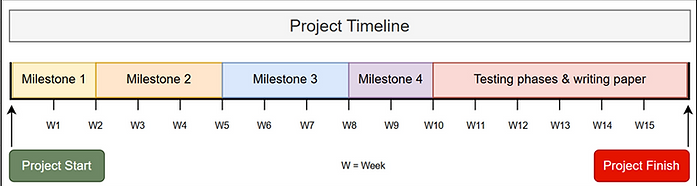
This project was developed over 16 weeks, and split into 4 major milestones:
1. Create a forward renderer supporting tessellation and compute with Dear ImGui integration.
2. Procedurally generate the geometry for blades of grass through the use of quadratic Bézier curves.
3. Implement applicable optimisation techniques as to not rely solely on a basic implementation.
4. Generate and monitor several performance and execution metrics for use in the research evaluation.
Within these 16 weeks, a 36-44 page document must be written, limiting development time. The 4 major milestones were planned to be implemented over a 10 week period, with the remaining 6 weeks to be for testing, evaluating, and writing.
The implementation was inspired by Jahrmann and Wimmer with their paper on Responsive Real-Time Grass Rendering for General 3D Scenes in 2017. Implemented in OpenGL, I felt that using its successor, Vulkan, would help innovate within this field. Their approach however, proposed several techniques that would be insufficient given the time period for development and so certain features were cut. For example, they proposed a physical model with grass blade collisions and recovery forces however, my implementation only considers wind forces. The displayed image showcases Jahrmann and Wimmer's final output of an oasis scene which inspired mine.

My biggest challenge throughout this module was to continue building my knowledge of shaders, which at the start of this module was quite minimal. Learning and understanding tessellation and compute shaders was a long process, but very worthwhile. The application started off as a compute-based particle system by Sascha Willems and evolved slowly but surely into the final scene displayed at the beginning of this page.
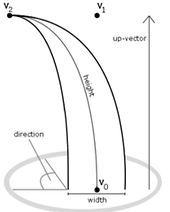
This is an image sourced from the paper by Jahrmann and Wimmer demonstrating the required data that constructs a grass blade. The 'skeleton' of each grass blade is represented as a quadratic Bézier curve containing some additional attributes:
-
Control point 1 and blade width
-
Control point 2 and blade height
-
Control point 3 and blade facing direction
-
Up vector and blade bend factor
This data can simply be packed into 4 vector4s with the xyz component representing a control point or vector and the w component representing a floating-point value. This packing also allows for more efficient memory alignment when creating buffers for the GPU.
To create and shape the geometry for the blade of grass, the application uses tessellation shaders. Grass blades are built as quads where their generated vertices are then displaced along the interpolation between a curve point for the left and right edges. This image describes the steps taken to go from a quadratic Bézier curve to a blade that is ready for shading. The first step shows the input and output to the vertex shader. The following steps show the results after the control and evaluation shaders.

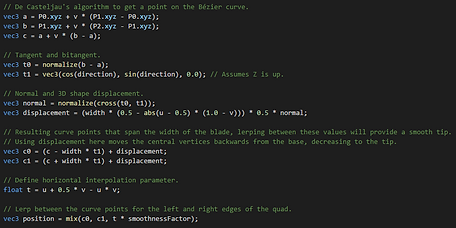
This is the code responsible for positioning the generated vertices from the tessellation primitive generator. Firstly the point along the Bézier curve needs to be evaluated and the necessary tangent, bitangent, and normal values to calculate a displacement value. From the base of the blade to its tip, the middle vertices are displaced along the normal vector of the blade, where the displacement decreases nearer the blade's tip. This is used to apply a 3D look to the blades and not have them completely 2D. Curve points for the left and right edges of the quad (seen in step 3 of the image above) are calculated where both values smoothly interpolation to the middle of the quad therefore, smoothing the top vertices into a smooth-tipped grass blade (as seen in step 4).
Additional to the grass tessellation, the terrain quad is also tessellated and samples a height map to give the scene a more natural environment. After failing many times to implement grass tessellation, terrain tessellation took a matter of minutes to implement. I also found that multiplying the output colour for the vertices by the sampled height provides a 'self-shadow'. This idea of self-shadowing is also applied to the grass blades, where the output colour is multiplied by the interpolation along the height of the blade to create a gradient from black at the base to more green at the tip.

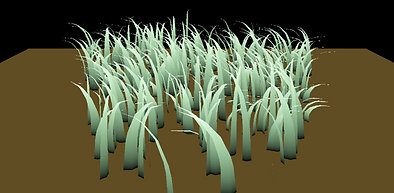
With the shaping of grass blades complete, I added the compute functionality which executes once per grass blade to apply 3D Perlin noise based wind to the control point representing the blade's tip. Additionally, compute-based frustum culling is performed to discard unnecessary work. The compute shader is dispatched before the graphics pipeline to ensure that:
-
the blades are within view and should be rendered
-
the positions of the control points are in their final positions before shaping
After spending some time implementing dynamic tessellation for the grass blades and some last minute changes, I achieved a complete procedural grass renderer using Vulkan. I am most proud of this project because, for the most part, I was left alone to create the application and I felt like my development time was quite limited in the grander scheme of things. I had full control to just make something and enjoy the process, and I did exactly that. I fell in love with this project and I wouldn't want to stop working on it and I had to remind myself of the true focus of this module, my research experiment.

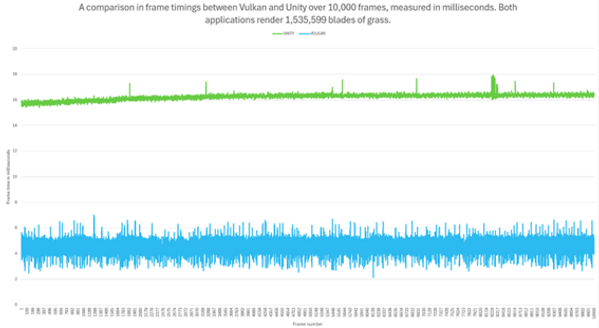
With my mind set to focus on the experimental aspect of this module, I found a comparable application in Unity by Aletuno which was the only package to match my design. This application required some tweaking since it featured additional shaders, an ocean, a grass paint brush, and additional terrain that had no grass. These needed removing to gain fair and accurate data for comparison. Additionally, Unity tries to lock their frame rates to 60fps so I spent a little time attempting to get a true performance value. I ran my test using a low and high instance count for the grass blades, once with 413,512 and once with 1,535,599 blades.
There are more graphs considered within the paper however, for simplicity I didn't want to overloaded this page.
Interestingly, Vulkan and Unity shared almost identical GPU usage yet Vulkan provided a significant frame time decrease of 271%. This implies that my results did support the question that using the GPU in a more explicit manner is much more effective than using an engine like Unity however, Unity does provide more consistency in their frame pacing.
Whilst Unity might provide a smoother user experience, Vulkan thrives in providing significantly low frame time in raw performance capabilities. Vulkan's timing inconsistencies may be fixable by increased synchronisation accuracies, more efficient memory usage, or additional optimisations since everything is at the responsibility of the developer and not the abstractions as provided by Unity.
For future work on this research, one could implement ambient occlusion, particle systems featuring flying insects or falling leaves, volumetric clouds, dynamic weather effects, and procedural terrain features such as valleys and rivers. Additionally, my approach doesn't consider the same physical behaviours of the grass blade like Jahrmann and Wimmer where they proposed gravity, recovery, and collision forces.
This project was graded a 81% overall.
You can read the full dissertation here.